Naming conventions
Microsoft has defined the C# naming conventions to create standards for all .NET developers. It mainly specifies the syntax of all elements to spread good practices.
Despites many naming conventions are existing, C# mainly used two of them:
- Pascal Case
- Camel Case
Pascal Case
The Pascal case syntax starts all words with a capital letter, and concatenates them.
Example: This is a goat => ThisIsAGoat
Globally, Pascal Case is used for anything that is externally accessible, i.e., by an element other than the current element: public
, internal
, protected
and protected internal
. In some cases, the private
element can also use this syntax, as we'll see later.
Let's get started with an exaustive list!
Namespaces and data types like class
, record
and struc
always use Pascal Case.
namespace MyNamespace
public class MyClass
internal class MyPrivateClass
public record MyRecord
internal record MyPrivateRecord
public struc MyStruc
internal struc MyPrivateStruc
Interfaces are completely the same, just add a capital I
as a prefix.
public interface IMyClass
It's really important to be able to differentiate it by name, so you don't have to dive deep into the code to check if each element is an interface or a data type.
Methods, regardless of accessibility, must also use the pascal case. This is the case where private
element are still in Pascal Case.
public void MyMethod()
private void MyMethod()
All class fields accessible from another class must also start with a capital letter, as if they were a method.
public class MyClass {
public string MyString;
protected string MyName {get; set;}
public event Action MyEvent;
}
Camel Case
The Camel Case syntax starts the first word with a lower case, the others with an upper case, and concatenates them.
Example: This is a goat => thisIsAGoat
It is used for private
and internal
fields and method parameters.
public class MyClass {
private string _myString;
private string _myName {get; set;}
void MyMethod(string firstParam, int secondParam)
}
If the field is static, add an small `s` before the `_`
If the field is thread, add an small `t` before the `_`
public class MyClass {
private static string s_myString;
[ThreadStatic]
private static t_myThread;
}
What's really the point making capital letter for accessible fields and small letter for internal fields ?
The answer is in the question. You no longer need to verify the field's implementation to understand if it's private or not, because the naming tells you the scope.
If you want to go further :

Define Visual Studio naming
The best way to make sure you follow the coding convention is to set Visual Studio to warn you if you forget it.
Thanks to us, we can define our own naming conventions in Text editor
> C#
> Code Style
> Naming
.
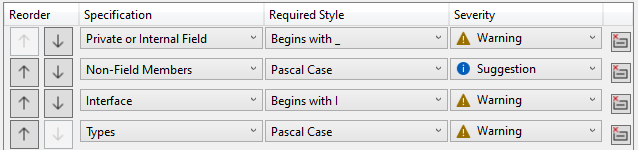
All existing conventions are displayed, but some like the s_
for private static fields are missing.
So let's add them.
First, create a new naming style that requires an s_
prefix.
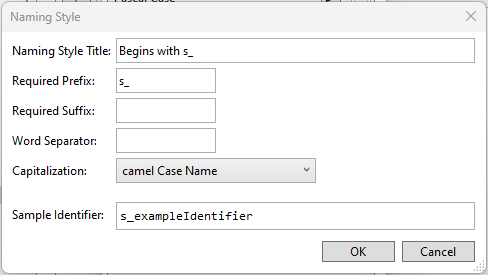
Second, add the required style to the Private or internal static field
specification. I prefer to set the severity to Warning
to quickly see omissions after a build.
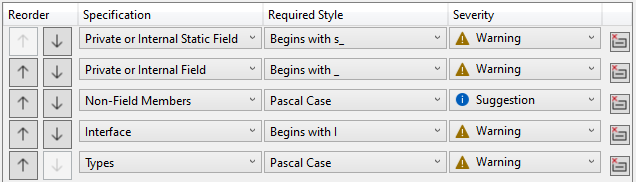
The list is not complete, it is up to you to add all the conventions.
That's it, you are now able to follow C# naming conventions 😎
Join the conversation.