C# has firmly established itself as a language of choice for many developers, especially when it comes to building high-performance applications.
This article explores the advanced features of C# that are pivotal in optimizing code performance, combining insights from both a theoretical and practical standpoint.
Exploring the Frontier of C# for Enhanced Performance
The continuous evolution of C# has introduced features that significantly impact how developers approach performance optimization.
Advanced features like Span, Memory, async streams, and ValueTask are not merely incremental updates; they represent a paradigm shift in efficient coding practices.
Span and Memory: Transforming Data Handling
Span and Memory have changed the game in data manipulation.
These features allow for more efficient memory management and data processing, crucial in scenarios involving large data sets or performance-critical applications.
Example: Optimizing Data Processing with Span
Consider an application that processes large data arrays, but only specific segments are relevant at any given time. Using Span, we can efficiently access and process these segments without unnecessary memory allocation.
public void OptimizeDataProcessing(byte[] data)
{
Span<byte> dataSpan = new Span<byte>(data);
var segment = dataSpan.Slice(start: 20, length: 100);
// Optimized processing of the segment...
}
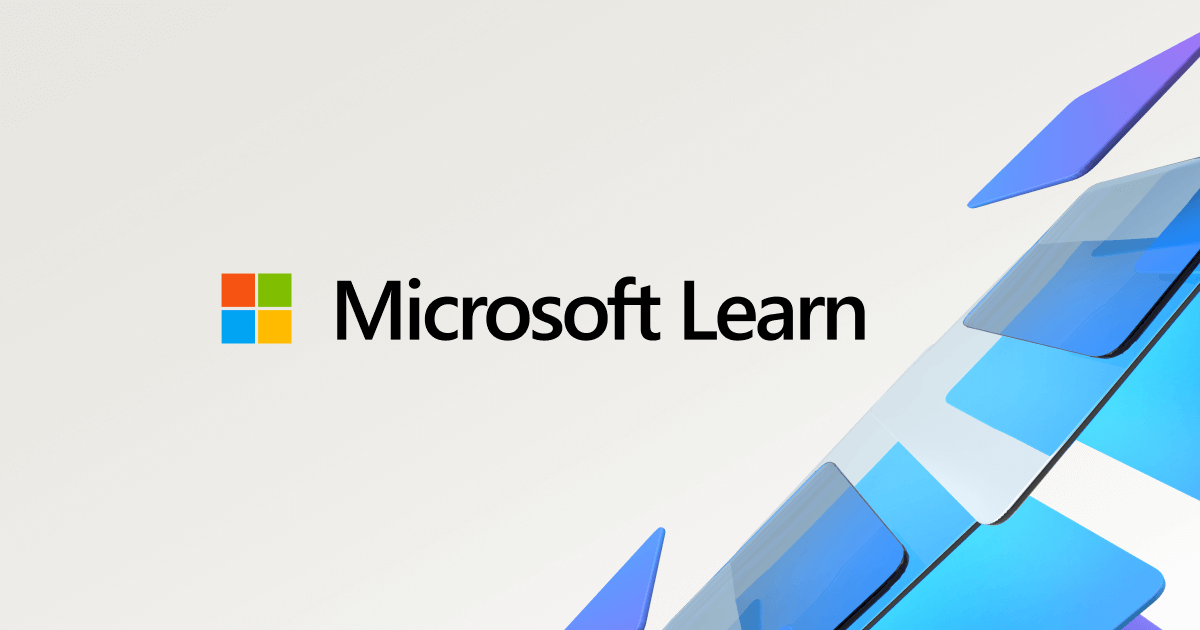
Example: Asynchronous File Processing with Memory
Memory<T>
is similar to Span<T>
, but it is more flexible in certain scenarios, especially when dealing with asynchronous operations. It's particularly useful for representing a contiguous region of memory, just like Span<T>
, but can be used on the heap and is compatible with async methods.
public async Task ProcessFileAsync(string inputFile, string outputFile)
{
const int bufferSize = 4096; // 4 KB buffer size
byte[] buffer = new byte[bufferSize];
Memory<byte> memoryBuffer = new Memory<byte>(buffer);
using (FileStream readStream = new FileStream(inputFile, FileMode.Open, FileAccess.Read, FileShare.Read, bufferSize, true))
{
int bytesRead;
while ((bytesRead = await readStream.ReadAsync(memoryBuffer)) > 0)
{
// Process the data in memoryBuffer here if needed
// Can be combined with Span method above
OptimizeDataProcessing(memoryBuffer.Slice(0, bytesRead));
}
}
}
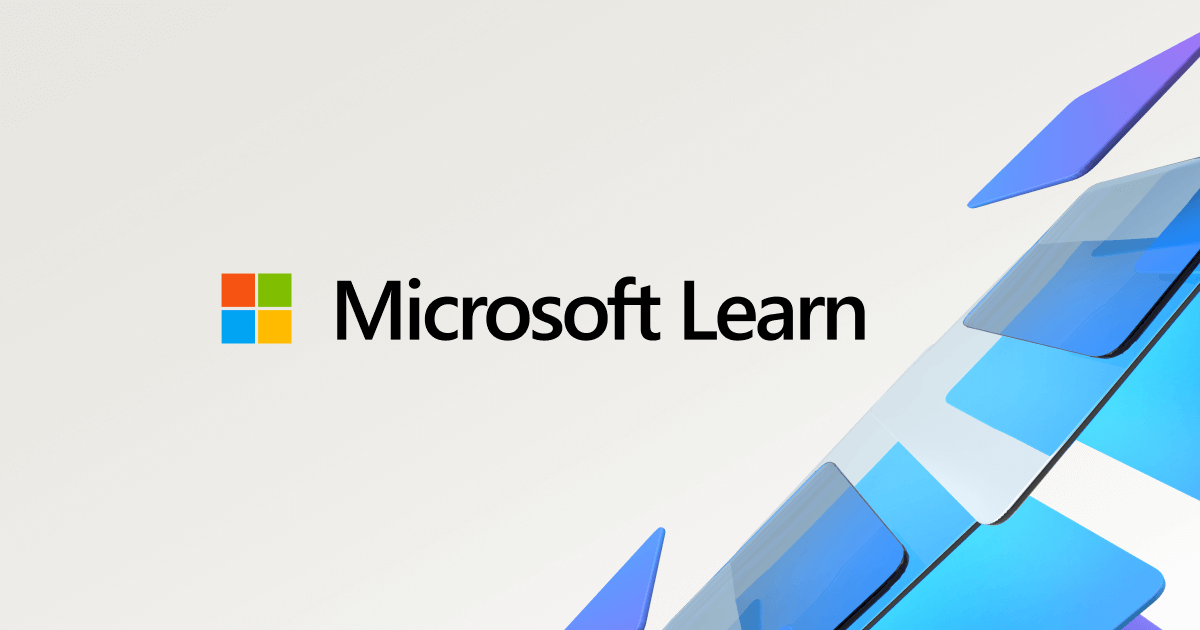
Async Streams: Elevating Asynchronous Operations
Async streams, a feature of C# 8.0, have revolutionized the way asynchronous data is handled, allowing for more efficient and readable code when dealing with asynchronous data sequences.
Example: Streamlining Asynchronous Data with Async Streams
In applications where data is asynchronously streamed, such as from a remote server, async streams simplify the handling of these data flows.
public async IAsyncEnumerable<int> EfficientDataStream()
{
for (int i = 0; i < 100; i++)
{
await Task.Delay(50); // Improved data streaming
yield return i;
}
}
public async Task HandleDataStream()
{
await foreach (var data in EfficientDataStream())
{
// Efficient asynchronous processing
}
}
We have combined the insightful theoretical perspectives and practical examples from both drafts to present a comprehensive view of optimizing code performance using C#'s advanced features.
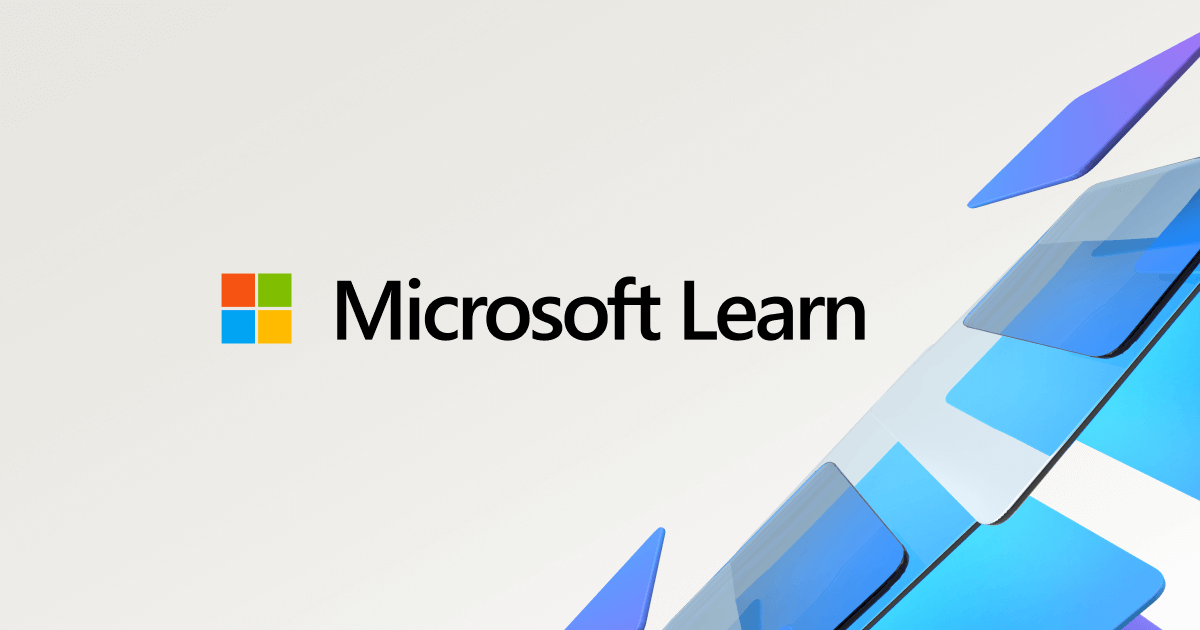
Summary
The article provides a detailed examination of how C# has become a preferred language for developing high-performance applications, focusing on its advanced features that enhance code optimization.
It delves into the impact of C#'s evolution on performance optimization, highlighting transformative features like Span, Memory, async streams, and ValueTask.
These are not just updates but represent a shift towards more efficient coding practices.
Have a goat day 🐐
Join the conversation.