Introduction
In modern distributed .NET applications, managing context across execution boundaries is a critical architectural concern. While both AsyncLocal<T>
and ThreadLocal<T>
provide mechanisms for maintaining contextual data, their implementations and use cases differ significantly in ways that impact system architecture, performance, and maintainability. This comprehensive guide explores both mechanisms through technical implementation details, architectural patterns, and real-world scenarios.
Core Implementation Mechanics
ThreadLocal: Thread-Bound Context Management
ThreadLocal<T>
creates thread-isolated variable instances, providing true thread-safety without explicit synchronization. Let's dive deeper into its implementation mechanics:
public class ThreadLocalManager
{
private static ThreadLocal<Dictionary<string, object>> _threadCache =
new ThreadLocal<Dictionary<string, object>>(
() => new Dictionary<string, object>(),
trackAllValues: false // Critical for memory management
);
public static void SetCacheValue(string key, object value)
{
if (!_threadCache.Value.ContainsKey("__metadata"))
{
_threadCache.Value["__metadata"] = new CacheMetadata
{
CreationTime = DateTimeOffset.UtcNow,
ThreadId = Thread.CurrentThread.ManagedThreadId
};
}
_threadCache.Value[key] = value;
}
}
In this example:
- The
ThreadLocal<Dictionary<string, object>>
ensures each thread maintains its own isolated dictionary instance - Setting
trackAllValues: false
prevents memory leaks by allowing garbage collection of values from terminated threads - The metadata entry provides crucial debugging information by tracking creation timestamps and thread ownership
- The implementation guarantees thread safety without locks, improving performance in high-concurrency scenarios
- The dictionary pattern allows for flexible storage of different data types while maintaining type safety through casting
AsyncLocal: Flow-Based Context Propagation
AsyncLocal<T>
leverages the execution context to maintain state across asynchronous boundaries. Here's a detailed look at its implementation:
public class AsyncContextManager
{
private static AsyncLocal<ExecutionContext> _context =
new AsyncLocal<ExecutionContext>(HandleContextChanged);
private static void HandleContextChanged(
AsyncLocalValueChangedArgs<ExecutionContext> args)
{
if (args.ThreadContextChanged)
{
var previous = args.PreviousValue?.ContextId ?? "none";
var current = args.CurrentValue?.ContextId ?? "none";
DiagnosticSource.Write("ContextSwitch", new
{
PreviousContext = previous,
CurrentContext = current,
Timestamp = DateTimeOffset.UtcNow
});
}
}
}
Key points about this implementation:
- The
AsyncLocal<T>
automatically maintains context across async/await boundaries without manual intervention - The
HandleContextChanged
callback provides real-time visibility into execution context transitions - The diagnostic events system enables detailed tracing of context flow through the application
- Context switches are automatically detected and logged, facilitating debugging of async operations
- The implementation handles null contexts gracefully, preventing NullReferenceException scenarios
Architectural Patterns and Considerations
Request Context Pattern
This pattern is particularly valuable in distributed systems. Let's examine its implementation in detail:
public class DistributedRequestContext : IDisposable
{
private static AsyncLocal<DistributedRequestContext> _current = new();
private readonly DistributedRequestContext _previousContext;
public string TraceId { get; }
public string ServiceId { get; }
public Dictionary<string, object> Baggage { get; }
public DateTimeOffset CreationTime { get; }
public DistributedRequestContext(string traceId, string serviceId)
{
TraceId = traceId;
ServiceId = serviceId;
Baggage = new Dictionary<string, object>();
CreationTime = DateTimeOffset.UtcNow;
_previousContext = _current.Value;
_current.Value = this;
}
public void Dispose()
{
_current.Value = _previousContext;
}
public static DistributedRequestContext Current => _current.Value
?? throw new InvalidOperationException("No active request context");
}
This implementation provides:
- Automatic context restoration using the disposable pattern, ensuring clean context management
- Thread-safe context storage leveraging AsyncLocal's built-in synchronization
- Support for nested context scenarios through careful tracking of previous contexts
- Immutable core properties (TraceId, ServiceId) to prevent context corruption
- Flexible baggage dictionary for additional context data that may vary by request
- Deterministic cleanup through the dispose pattern, preventing context leaks
- Safe access to the current context with clear error messaging
Resource Management Pattern
The ResourceScope implementation demonstrates proper resource management in async contexts:
public class ResourceScope : IAsyncDisposable
{
private static AsyncLocal<Stack<IDisposable>> _resourceStack =
new AsyncLocal<Stack<IDisposable>>(() => new Stack<IDisposable>());
private readonly IDisposable _resource;
private readonly DiagnosticSource _diagnostics;
public ResourceScope(IDisposable resource)
{
_resource = resource;
_diagnostics = new DiagnosticListener("ResourceScope");
_resourceStack.Value ??= new Stack<IDisposable>();
_resourceStack.Value.Push(resource);
_diagnostics.Write("ResourceAcquired", new
{
ResourceType = resource.GetType().Name,
StackDepth = _resourceStack.Value.Count
});
}
public async ValueTask DisposeAsync()
{
if (_resourceStack.Value?.TryPop(out var resource) == true)
{
if (resource is IAsyncDisposable asyncDisposable)
{
await asyncDisposable.DisposeAsync();
}
else
{
resource.Dispose();
}
_diagnostics.Write("ResourceReleased", new
{
ResourceType = resource.GetType().Name,
RemainingStackDepth = _resourceStack.Value.Count
});
}
}
}
Key features of this implementation:
- Unified handling of both synchronous IDisposable and asynchronous IAsyncDisposable resources
- Thread-safe resource tracking using AsyncLocal<Stack
> - Comprehensive diagnostic events for resource lifecycle monitoring
- Proper management of nested resource scopes through stack-based tracking
- Guaranteed resource cleanup through structured async disposal pattern
- Detailed telemetry for resource acquisition and release operations
Performance Implications and Optimization
Memory Impact Analysis
The performance analysis implementation provides detailed insights into memory usage. Note that ThreadMetrics
represents per-thread performance data including the thread ID and bytes processed, while PerformanceMetrics
aggregates these metrics across all threads and includes total memory usage:
public class PerformanceAnalysis
{
private static ThreadLocal<MemoryStream> _threadBuffer =
new ThreadLocal<MemoryStream>(() => new MemoryStream(1024));
private static AsyncLocal<MemoryStream> _asyncBuffer =
new AsyncLocal<MemoryStream>();
public static async Task<PerformanceMetrics> MeasureMemoryImpactAsync(
int concurrentOperations,
int operationsPerThread)
{
var startMemory = GC.GetTotalMemory(true);
var metrics = new ConcurrentDictionary<int, ThreadMetrics>();
var tasks = Enumerable.Range(0, concurrentOperations)
.Select(async i =>
{
var threadMetrics = new ThreadMetrics
{
ThreadId = Thread.CurrentThread.ManagedThreadId
};
for (int j = 0; j < operationsPerThread; j++)
{
using (var scope = new ResourceScope(new MemoryStream(1024)))
{
_threadBuffer.Value.Position = 0;
_asyncBuffer.Value = new MemoryStream(1024);
await ProcessDataAsync();
threadMetrics.BytesProcessed += _threadBuffer.Value.Position;
}
}
metrics[Thread.CurrentThread.ManagedThreadId] = threadMetrics;
});
await Task.WhenAll(tasks);
return new PerformanceMetrics
{
TotalMemoryUsed = GC.GetTotalMemory(false) - startMemory,
ThreadMetrics = metrics.Values.ToList()
};
}
}
This implementation:
- Compares memory allocation patterns between ThreadLocal and AsyncLocal buffers
- Tracks granular performance metrics at both thread and application levels
- Uses concurrent collections to safely aggregate metrics across threads
- Implements proper resource cleanup through using statements
- Provides accurate memory delta measurements using GC.GetTotalMemory
- Maintains thread-safety through concurrent data structures
Critical Performance Considerations
- Thread Pool Impact
public class ThreadPoolOptimization
{
private static ThreadLocal<ObjectPool<byte[]>> _bufferPool =
new ThreadLocal<ObjectPool<byte[]>>(() =>
new ObjectPool<byte[]>(() => new byte[4096], 50));
public async Task ProcessLargeDataSetAsync(Stream data)
{
var buffer = _bufferPool.Value.Rent();
try
{
await ProcessDataChunkAsync(data, buffer);
}
finally
{
_bufferPool.Value.Return(buffer);
}
}
}
This optimization:
- Creates efficient thread-specific buffer pools to minimize allocation pressure
- Implements buffer recycling to reduce garbage collection overhead
- Maintains strict thread isolation for optimal performance
- Uses a fixed buffer size to prevent memory fragmentation
- Implements proper buffer return mechanism to prevent leaks
- Leverages try-finally pattern for guaranteed buffer return
- Context Switch Overhead
public class ContextSwitchAnalysis
{
private static AsyncLocal<ExecutionContext> _executionContext =
new AsyncLocal<ExecutionContext>();
public async Task MeasureContextSwitchOverheadAsync()
{
var sw = new Stopwatch();
var metrics = new List<TimeSpan>();
for (int i = 0; i < 1000; i++)
{
sw.Restart();
await Task.Run(() =>
{
_executionContext.Value = new ExecutionContext
{
OperationId = Guid.NewGuid()
};
});
metrics.Add(sw.Elapsed);
}
var averageSwitchTime = TimeSpan.FromTicks(
(long)metrics.Average(t => t.Ticks));
}
}
This analysis:
- Measures precise context switch timing using high-resolution Stopwatch
- Collects comprehensive timing metrics across multiple iterations
- Calculates accurate average switch duration using tick-level precision
- Simulates real-world async context switching scenarios
- Provides statistical insights into context switch overhead
- Enables identification of performance bottlenecks in async operations
Integration with Distributed Systems
Distributed Tracing Integration
public class DistributedTraceManager
{
private static AsyncLocal<TraceContext> _traceContext =
new AsyncLocal<TraceContext>();
private static ThreadLocal<DiagnosticSource> _diagnostics =
new ThreadLocal<DiagnosticSource>(() =>
new DiagnosticListener($"Trace.{Thread.CurrentThread.ManagedThreadId}"));
public static async Task<T> TraceOperationAsync<T>(
string operationName,
Dictionary<string, string> tags,
Func<Task<T>> operation)
{
var parentContext = _traceContext.Value;
_traceContext.Value = new TraceContext
{
OperationId = Guid.NewGuid(),
ParentId = parentContext?.OperationId,
OperationName = operationName,
Tags = new Dictionary<string, string>(tags),
StartTime = DateTimeOffset.UtcNow
};
try
{
_diagnostics.Value?.Write("OperationStart", _traceContext.Value);
return await operation();
}
catch (Exception ex)
{
_traceContext.Value.Error = ex;
throw;
}
finally
{
_traceContext.Value.EndTime = DateTimeOffset.UtcNow;
_diagnostics.Value?.Write("OperationEnd", _traceContext.Value);
_traceContext.Value = parentContext;
}
}
}
This implementation provides:
- Hierarchical trace context tracking
- Automatic parent-child relationship management
- Error tracking and propagation
- Timing information for operations
- Thread-safe diagnostic logging
- Context restoration on completion
Feature Comparison Matrix
Aspect | ThreadLocal |
AsyncLocal |
---|---|---|
Architectural Fit | Traditional multi-threaded | Modern async applications |
Scaling Pattern | Vertical (thread-based) | Horizontal (context-based) |
Resource Management | Manual | Automatic with scope |
Integration Complexity | Higher | Lower |
Distributed Tracing | Limited support | Native support |
Memory Scalability | Linear with threads | Linear with contexts |
Best Practices and Design Patterns
Choose ThreadLocal When:
- Working with thread-specific resources that require explicit cleanup
- Performance is critical and context doesn't need to flow across async boundaries
- Implementing thread-specific caching mechanisms
Choose AsyncLocal When:
- Building distributed systems with context propagation requirements
- Implementing cross-cutting concerns like logging and tracing
- Working with modern async/await patterns
- Dealing with microservices architectures
Conclusion
The choice between ThreadLocal<T>
and AsyncLocal<T>
extends beyond simple technical differences to fundamental architectural considerations. Modern .NET applications, especially those built on microservices architectures, benefit significantly from AsyncLocal<T>
's superior context propagation capabilities. However, ThreadLocal<T>
remains valuable for specific performance-critical scenarios where thread-bound data is appropriate.
Understanding these mechanisms' implementation details, performance implications, and architectural patterns is crucial for building robust, maintainable, and scalable .NET applications.
To go further:
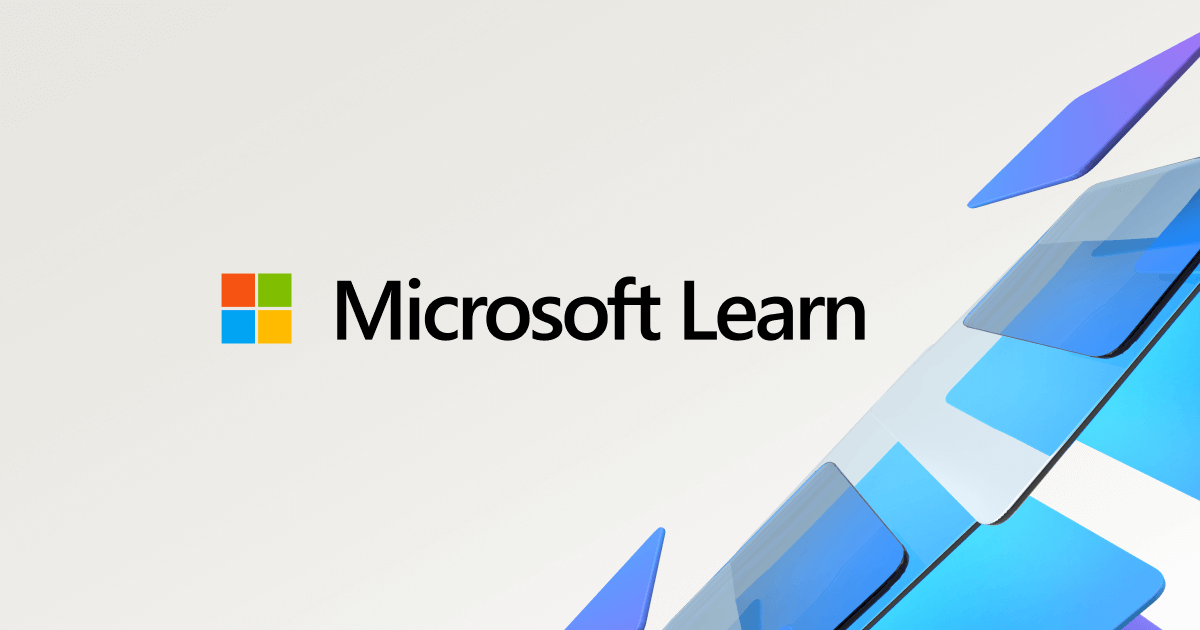
and
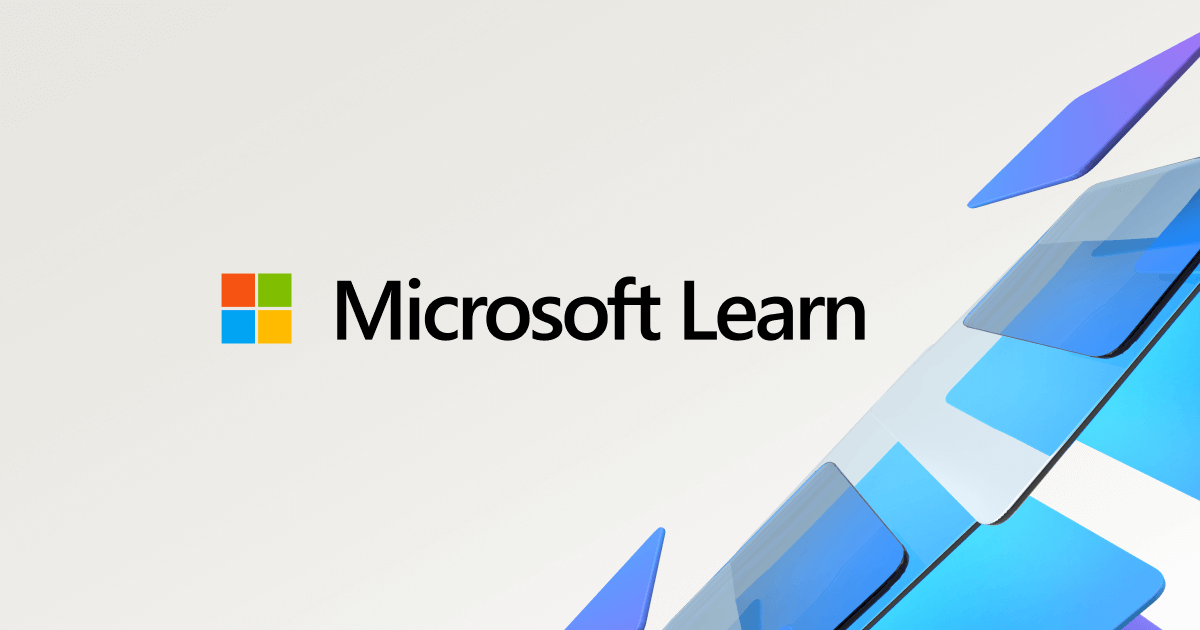
Have a goat day 🐐
Join the conversation.