In the world of software development, optimizing resource usage and performance is crucial. When it comes to object initialization, performing it upfront can sometimes lead to unnecessary resource consumption.
Ever wondered how to make your software development more efficient?
Well, let me tell you about a nifty trick called lazy initialization. It's all about delaying the creation of objects until you really need them. And guess what? In C#, we have the perfect tool for that – the amazing Lazy<T> class.
In this article, we'll take a deep dive into Lazy<T> and discover its awesome features and benefits in C#. You'll learn how it simplifies delayed initialization and takes your resource management skills to the next level.
So, let's dive into the world of Lazy<T> and unlock its potential in optimizing your C# projects.
Understanding Lazy Initialization
Let's start with the exact definition of Microsoft:
Lazy initialization of an object means that its creation is deferred until it is first used
- Microsoft
Lazy initialization approach offers several benefits, such as optimizing resource utilization and improving performance. By implementing it, you can avoid unnecessary overhead and delays in our applications.
One common scenario where lazy initialization proves useful is when dealing with expensive object creation or computations. For example, imagine a scenario where you have a complex and resource-intensive object that is not always required during the execution of your program. By lazily initializing that object, you can postpone its creation until it is explicitly requested, reducing unnecessary resource consumption.
In C#, the Lazy<T> class provides a convenient and efficient way to implement lazy initialization. This generic class allows us to delay the creation of an object until it is accessed for the first time. Lazy<T> ensures that the initialization logic is executed only once and that subsequent requests for the object return the previously created instance.
Exploring the Lazy<T> Class
The Lazy<T> class in C# is a versatile tool for implementing lazy initialization. It takes advantage of the type parameter T to ensure type safety and provides various constructors and properties to customize its behavior.
An important aspect of Lazy<T> is the LazyThreadSafetyMode
enum, which determines how the class handles thread safety in concurrent environments.
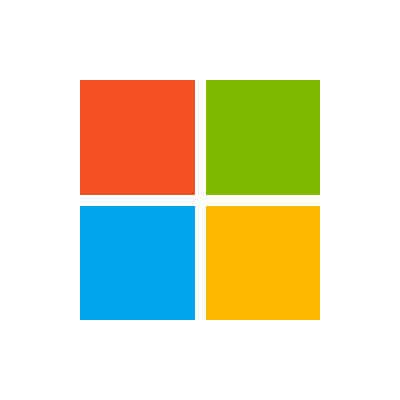
By choosing the appropriate security mode, such as PublicationOnly
or ExecutionAndPublication
, you can control how the Lazy instance handles multiple threads accessing the object simultaneously.
Lazy<T> also offers 2 properties:
IsValueCreated
which allows you to check whether the object has already been createdValue
which retrieves the lazily initialized object.
These properties allow you to manage the initialization process and access the resulting object when needed.
Practical Examples
Let's get back to our goats!
Consider a web application that needs to retrieve goat profile images from a remote server. Loading all the images upfront can lead to unnecessary network requests and increased latency. By using Lazy<T>, we can delay the image retrieval until the goat actually accesses their profile.
public class GoatProfile
{
private readonly Lazy<Image> _lazyProfileImage;
public GoatProfile()
{
_lazyProfileImage = new Lazy<Image>(() => RetrieveProfileImage());
}
public Image ProfileImage => _lazyProfileImage.Value;
private Image RetrieveProfileImage()
{
// Perform the network request to retrieve the goat's profile image
// Return the retrieved image
}
}
In this example, the GoatProfile
class incorporates Lazy<T> to lazily load the user's profile image. The first access to the ProfileImage
property triggers the retrieval process through the lambda expression, while subsequent requests return the cached image. This approach optimizes network utilization by only fetching the image when it is explicitly requested.
Summary
Incorporating the Lazy<T> class in C# enables you to embrace lazy initialization and optimize resource usage.
By deferring object creation until necessary, you enhance performance and reduce unnecessary overhead. It can strike a balance between responsiveness and resource utilization, ultimately delivering smoother and more efficient applications.
I discovered this feature recently, and I can see the benefits, especially for objects that build external connections when they're created (with RabbitMq, Redis) and then instantiate them only the first time they're used.
To go further, you can read the Microsoft documentation :
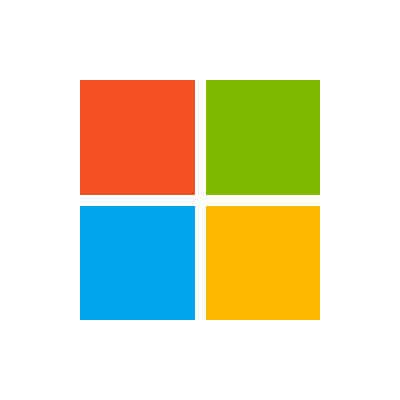
Have a goat day 🐐
Join the conversation.